지난 포스팅과 이어지는 3번째 Notification 포스팅이다.
Notification 그룹화에 대해 알아보자.
Group Notification ?
Android 7 (API LEVEL 24) 부터 지원되며
동일한 그룹 식별자를 가진 알림을 하나의 그룹으로 묶어서 드롭다운 형식으로 제공한다.
위 사진을 보면 한 번에 이해가 될 것이다.
우리가 지난 포스팅까지 사용해봤던 방법은 다음과 같은 알림이었다.
동일한 Notification ID를 가진 알림은 여러 번 발생하더라도
여러 개가 쌓이지 않고 하나의 알림이 계속 업데이트 되는 방식이다.
그리고, 각기 다른 Notification ID를 가진 알림은 다음과 같이 여러 개의 알림이 쌓인다.
하지만 위와 같은 알림은 알림 드로어 뿐만 아니라 상태 표시줄에서도 지저분한 느낌을 준다.
Automatic Grouping : 자동 그룹화
안드로이드에서는 시스템적으로 자동 그룹화를 지원한다.
지난 포스팅에서 Notification ID를 구분하여 여러개의 알림을 발생시켜 보았다.
위와 같은 방식으로 알림을 4개 이상 발생시키면 다음과 같이 자동 그룹화된다.
별도로 알림 그룹화 코드를 작성하지 않아도 4개의 알림이 그룹화 된 모습이다.
알림 우측 상단의 드롭다운 아이콘을 누르면 다음과 같이 펼쳐진다.
하지만 이와 같은 자동 그룹화 동작은 일부 장치 유형이나 설정에 따라 동작하지 않을 수 있다.
또한, 개발자의 의도대로 그룹화되지 않을 수 있다.
그렇기에 수동으로 그룹화 동작을 구현하는 것을 권장한다.
사용해보자
✅ Notification 생성 시 setGroup() 함수로 그룹 지정
val groupKey = "Oscar Group"
val notification = NotificationCompat.Builder(applicationContext, channelId).apply {
setSmallIcon(R.drawable.ic_launcher_foreground)
...
setGroup(groupKey)
}.build()
그룹화 하고싶은 Notification 객체에 groupKey를 지정해줘야 한다.
당연히 groupKey가 동일한 알림끼리 그룹화된다.
✅ 그룹 전용 Notification 생성
공식 문서에서는 그룹 알림이 아닌, 요약 알림이라 칭한다. (Summary Notification)
하지만 본 포스팅에서는 그룹 알림이라 부르겠다.
val groupNotification = NotificationCompat.Builder(applicationContext, channelId).apply {
setSmallIcon(R.drawable.ic_launcher_foreground)
setGroupSummary(true)
setGroup(groupKey)
}.build()
그룹 알림의 필수 요소만 작성해주었다.
● setGroupSummary(true)
해당 알림을 그룹 알림으로 지정한다.
● setGroup(groupKey)
그룹화 할 알림들과 동일한 groupKey로 지정해줘야 한다.
Title, Body 등은 옵셔널이기에, 상황에 맞게 추가하면 된다.
✅ 알림 발생
notificationManager.apply {
notify(notificationId, notification)
notify(groupNotificationId, groupNotification)
}
// Notification ID 예시
notificationId = 1, 2, 3 ... // 알림 식별자로 사용
groupNotificationId = 99999 // 그룹화 할 단위마다 고정값으로 사용
발생시킬 기본 알림과 그룹 알림을 함께 호출해줘야 한다.
Notification ID의 경우, 기본 알림은 각각 다르게 지정해야 여러 개의 알림이 쌓인다고 설명했었다.
하지만 그룹 알림의 Notification ID는 그룹 단위로 동일한 ID를 사용해야 한다.
✅ 실행 결과
알림을 4개 이상 발생시키지 않아도 그룹화에 성공한 모습이다.
✅ 알림 그룹화 전체 코드
// 초기 변수 세팅
val channelId = "Notification Channel"
val channelName = "Channel Name"
val title = "Test Notification"
val groupKey = "Oscar Group"
val groupNotificationId = 99999
// 버튼 클릭 시 알림 3개 발생
notiButton.setOnClickListener {
for (i in 1 .. 3) setNoti(i)
}
// 알림 세팅 & 발생 함수
private fun setNoti(count: Int) {
val body = "$count 번 째 알림입니다."
// Notification Manager 세팅
val notificationManager = NotificationManagerCompat.from(applicationContext)
if (notificationManager.getNotificationChannel(channelId) == null) {
val channel = NotificationChannel(channelId, channelName, NotificationManager.IMPORTANCE_HIGH)
notificationManager.createNotificationChannel(channel)
}
// Notification 세팅
val notification = NotificationCompat.Builder(applicationContext, channelId).apply {
setSmallIcon(R.drawable.ic_launcher_foreground)
setContentTitle(title)
setContentText(body)
setGroup(groupKey)
}.build()
// Group Notification 세팅
val groupNotification = NotificationCompat.Builder(applicationContext, channelId).apply {
setSmallIcon(R.drawable.ic_launcher_foreground)
setGroup(groupKey)
setGroupSummary(true)
}.build()
// 알림 발생
notificationManager.apply {
notify(count, notification)
notify(groupNotificationId, groupNotification)
}
}
3차례에 걸쳐 Notification에 대해 알아보았다.
그만큼 다룰 내용이 많았던 것 같다.
그래도 아직 Notification Action 등 못 다룬 부분이 더 많다...
다음 프로젝트에서 Notification을 더 사용할 일이 생기면 그 때 포스팅 할 예정이다.
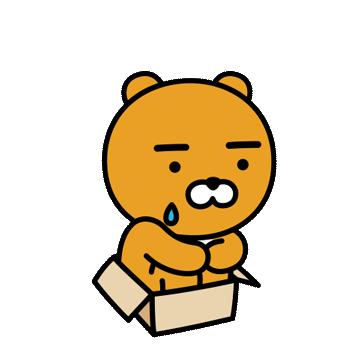